How to Optimize React App Performance Using useMemo & useCallback Hooks in 2023
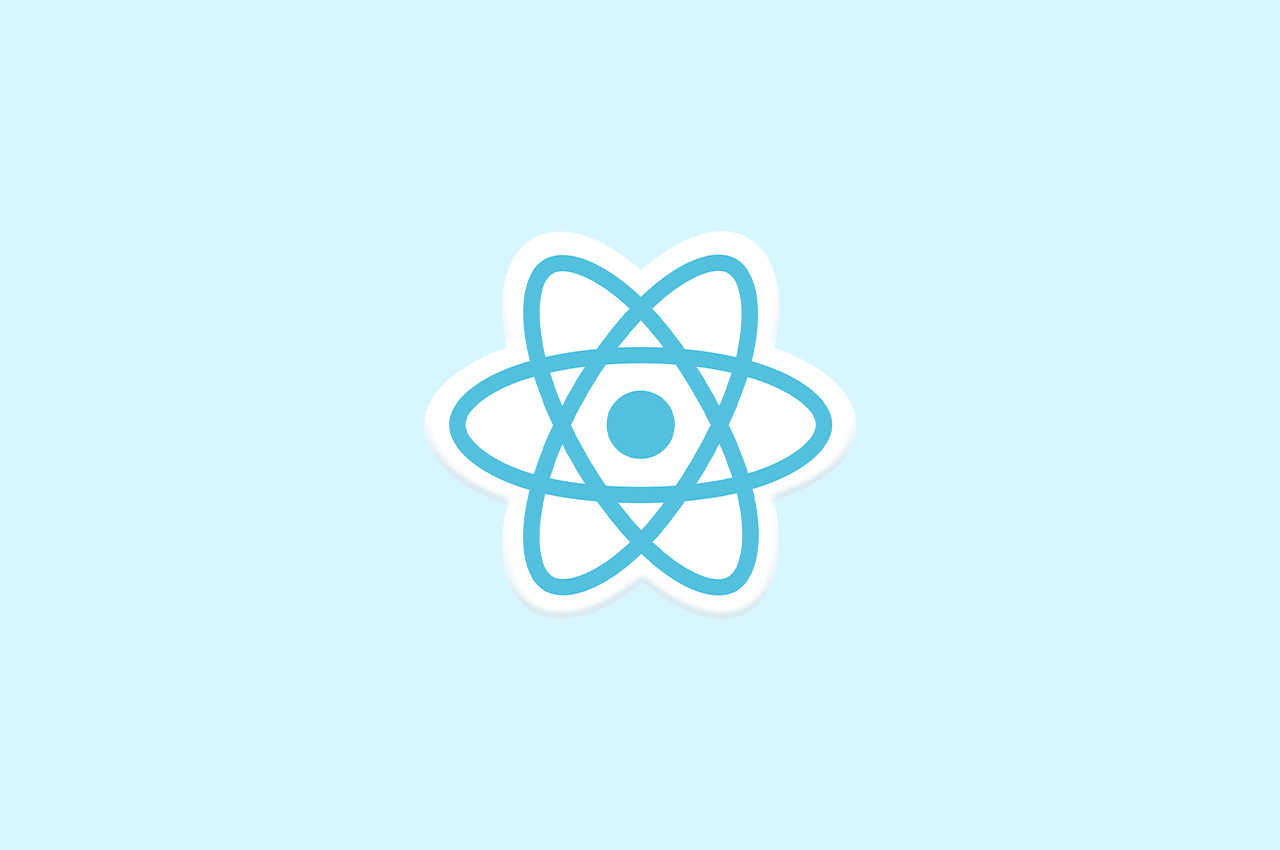
React is known for its efficient rendering and re-rendering mechanisms, but as your applications grow, performance optimizations become increasingly important. Two essential hooks, useMemo and useCallback, play a crucial role in optimizing your React components by implementing memoization.
In this blog, we’ll explore the significance of memoization, understand how useMemo and useCallback work, and discover how to leverage them to improve the performance of your React applications.
Understanding Memoization
Memoization is a technique used to optimize expensive calculations or function calls by caching their results and returning the cached result when the same input is encountered again. In React, this translates into optimizing the rendering of components to avoid unnecessary re-renders.
To read more about useEffect Hook & how to manage side effects in React, refer to our blog What is UseEffect Hook & How to Manage Side Effects in React
The useMemo Hook
The useMemo hook is a powerful tool for optimizing the computation of values that depend on one or more props or state variables. It takes two arguments: a function and an array of dependencies. The function contains the computation you want to memoize, and the dependencies determine when the memoized value should be recalculated.
Let’s look at an example where we use useMemo to compute a factorial:
import React, { useState, useMemo } from 'react';
function FactorialCalculator({ number }) {
const calculateFactorial = (n) => {
if (n === 0) return 1;
return n * calculateFactorial(n - 1);
};
const factorial = useMemo(() => calculateFactorial(number), [number]);
return (
<div>
<p>Factorial of {number} is {factorial}</p>
</div>
);
}
In this example, calculateFactorial is an expensive computation. We use useMemo to memoize the result based on the number prop. The factorial will only be recalculated when the number prop changes, avoiding unnecessary computations on each render.
The useCallback Hook
The useCallback hook is used for memoizing functions. It’s particularly useful when you want to prevent functions from being recreated on every render, which can lead to unnecessary re-renders of child components that depend on these functions.
Consider a scenario where you have a child component that receives a callback function as a prop. Without useCallback, this callback would be recreated every time the parent component re-renders, potentially causing the child component to re-render unnecessarily.
Let’s see how useCallback can help:
import React, { useState, useCallback } from 'react';
function ParentComponent() {
const [count, setCount] = useState(0);
const handleClick = useCallback(() => {
setCount(count + 1);
}, [count]);
return (
<div>
<p>Count: {count}</p>
<ChildComponent onClick={handleClick} />
</div>
);
}
function ChildComponent({ onClick }) {
return (
<button onClick={onClick}>
Increment Count
</button>
);
}
In this example, handleClick is memoized using useCallback. It depends on the count state variable, so we include [count] in the dependency array. This guarantees that handleClick is regenerated only when there is a change in the count variable. Without useCallback, handleClick would be recreated on every render of ParentComponent.
When to Use useMemo and useCallback
- Use useMemo when: You want to memoize the result of an expensive computation based on props or state, and you want to recalculate it only when the dependencies change.
- Use useCallback when: You need to cache a function to prevent child components from re-rendering unnecessarily, especially when those components depend on it, and you want to recalculate it only when the dependencies change.
Performance Considerations
While useMemo and useCallback can significantly improve performance by reducing unnecessary re-renders, it’s important not to overuse them. Memoization comes with a trade-off in terms of memory usage, as it caches values and functions. Therefore, use these hooks judiciously for parts of your components where performance gains are critical.
To read more about what is React, refer to our blog What is React? A Beginner’s Guide to JavaScript Library
Conclusion
In React, optimizing performance is an ongoing process, and memoization is a powerful technique to achieve that. The useMemo and useCallback hooks are valuable tools that enable you to memoize values and functions, respectively, improving the efficiency of your React applications. By strategically applying these hooks to compute values and memoize functions, you can ensure that your components render efficiently and respond to changes in props and state with precision.