What is the Use of Serializers in Django Rest Framework [DRF] in 2024
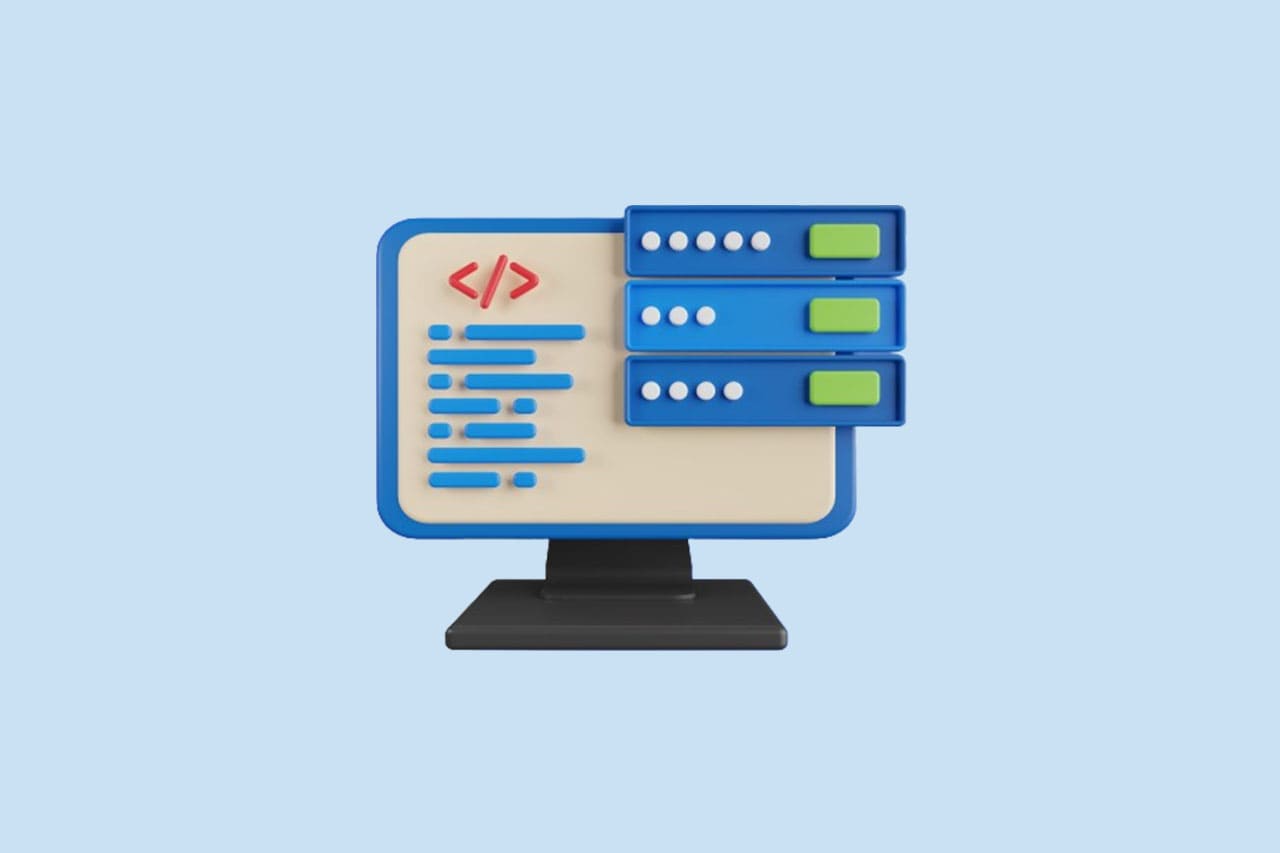
Django Rest Framework (DRF) serves as a robust and adaptable toolkit designed for constructing Web APIs within Django applications. Among its integral components, serializers hold a pivotal role in the conversion of intricate data types, such as Django models or querysets, into Python data types. This transformation facilitates seamless rendering into various content types, including JSON and others. Essentially, serializers act as a fundamental element within DRF, streamlining the process of translating complex data structures into formats easily comprehensible by external applications or services, promoting effective communication and interoperability.
In this blog post, we’ll delve into the world of serializers in Django Rest Framework, exploring their purpose, usage, and some advanced features.
What is a Serializer?
Django Rest Framework (DRF), a serializer functions as a tool to transform intricate data types, like Django models or querysets, into Python data types. This conversion is instrumental in facilitating the straightforward rendering of data into JSON format. Essentially, a serializer acts as a mechanism within DRF, ensuring the smooth conversion of complex data structures into Python types, which can then be easily translated into JSON, promoting simplicity and compatibility in data representation. The process of converting complex data into a format suitable for rendering or parsing is known as serialization and deserialization, respectively.
In simpler terms, serializers allow you to convert data from Python objects into a format that can be easily rendered into JSON or parsed from incoming JSON requests.
This aspect is particularly crucial when dealing with APIs, as the standard practice involves the exchange of data in JSON format. This necessity underscores the importance of serializers in Django Rest Framework, as they enable the seamless translation of intricate data types into Python representations, which can then be effortlessly converted into JSON. In essence, serializers play a pivotal role in ensuring compatibility and effectiveness in the communication of data through APIs.
Basic Usage of Serializers
Creating a Serializer
To use a serializer, you first need to define one. Serializers in DRF are similar to Django forms and models. Let’s create a simple serializer for a hypothetical Book model:
# serializers.py from rest_framework import serializers from .models import Book class BookSerializer(serializers.ModelSerializer): class Meta: model = Book fields = '__all__'
In this example, BookSerializer is inheriting from serializers.ModelSerializer, which is a shortcut for creating serializers for Django models. The fields = ‘__all__’ line indicates that all fields from the Book model should be included in the serialization.
Using the serializer
After establishing your serializer, you can employ it for both serializing and deserializing data. In a view, the utilization of the serializer typically involves actions similar to the following:
# views.py from rest_framework.response import Response from rest_framework.views import APIView from .models import Book from .serializers import BookSerializer class BookAPIView(APIView): def get(self, request): books = Book.objects.all() serializer = BookSerializer(books, many=True) return Response(serializer.data)
In this example, we use the BookSerializer to serialize a queryset of Book objects. The resulting data can then be used to respond to an API request.
Serialization and Deserialization
Serialization
Serialization is the procedure of transforming intricate data types into a format suitable for rendering. Within the context of Django Rest Framework (DRF), this commonly entails converting Django models or querysets into Python data types. These Python data types can then be effortlessly rendered into various content types, such as JSON or others. The BookSerializer we created earlier handles the serialization of Book objects. When we call serializer.data, it returns a dictionary representing the serialized data.
# Example serialized data { 'id': 1, 'title': 'The Great Gatsby', 'author': 'F. Scott Fitzgerald', 'published_date': '1925-04-10', 'isbn': '9780743273565', 'page_count': 180, 'cover_image': 'http://example.com/gatsby.jpg', }
Deserialization
Deserialization is the reverse process of converting incoming data, usually in JSON format, into complex data types like Django models. DRF serializers handle this as well.
Let’s modify our BookAPIView to handle POST requests for creating new books:
# views.py from rest_framework import status from rest_framework.response import Response from rest_framework.views import APIView from .models import Book from .serializers import BookSerializer class BookAPIView(APIView): def get(self, request): books = Book.objects.all() serializer = BookSerializer(books, many=True) return Response(serializer.data) def post(self, request): serializer = BookSerializer(data=request.data) if serializer.is_valid(): serializer.save() return Response(serializer.data, status=status.HTTP_201_CREATED) return Response(serializer.errors, status=status.HTTP_400_BAD_REQUEST)
In this example, when a POST request is received, we use the BookSerializer to validate and save the incoming data. If the data is valid, a new Book object is created, and the serialized data of the created object is returned in the API response.
Validation with Serializers
Serializers in DRF provide a powerful mechanism for data validation. The validation process ensures that the incoming data conforms to the expected structure and meets specific criteria.
Let’s enhance our BookSerializer to include validation:
# serializers.py from rest_framework import serializers from .models import Book class BookSerializer(serializers.ModelSerializer): class Meta: model = Book fields = '__all__' def validate_published_date(self, value): """ Validate that the published date is not in the future. """ from datetime import date if value > date.today(): raise serializers.ValidationError("Published date cannot be in the future.") return value
In this example, we’ve added a custom validation method validate_published_date to the BookSerializer. This method checks if the published date is in the future and raises serializers.ValidationError if the condition is not met.
Nesting Serializers
Serializers in DRF support nested structures, allowing you to represent complex relationships between models. Let’s consider a scenario where each book can have multiple reviews, and we want to include reviews in the serialization of a book:
# serializers.py class ReviewSerializer(serializers.ModelSerializer): class Meta: model = Review fields = '__all__' class BookSerializer(serializers.ModelSerializer): reviews = ReviewSerializer(many=True, read_only=True) class Meta: model = Book fields = '__all__'
In this example, the BookSerializer includes a nested ReviewSerializer. The reviews field is set to ReviewSerializer(many=True, read_only=True), indicating that it can represent multiple reviews and is read-only.
When a Book object is serialized, the associated reviews will be included in the output:
{ "id": 1, "title": "The Great Gatsby", "author": "F. Scott Fitzgerald", "published_date": "1925-04-10", "isbn": "9780743273565", "page_count": 180, "cover_image": "http://example.com/gatsby.jpg", "reviews": [ { "id": 1, "rating": 5, "comment": "A timeless classic." }, { "id": 2, "rating": 4, "comment": "Well-written, but the ending left me wanting more." } ] }
Customizing Serialization Output
DRF serializers provide flexibility in customizing the output format. You can override methods to control how individual fields are represented in the serialized data.
Custom Field Representation
Let’s modify our BookSerializer to represent the published_date in a different format:
# serializers.py class BookSerializer(serializers.ModelSerializer): published_date = serializers.DateField(format="%Y-%m-%d") class Meta: model = Book fields = '__all__'
In this example, we override the representation of the published_date field by specifying the format attribute in the DateField. This ensures that the date is serialized in the specified format.
Customizing Entire Serialization Output
You can also customize the entire serialized representation of an object by overriding the to_representation method:
# serializers.py class BookSerializer(serializers.ModelSerializer): class Meta: model = Book fields = '__all__' def to_representation(self, instance): representation = super().to_representation(instance) representation['title'] = representation['title'].upper() return representation
In this example, we use the to_representation method to modify the serialized output. In this case, we convert the title to uppercase. This method allows you to have fine-grained control over the serialized representation.
To read more about creating a chat-bot using Django DRF, refer to our blog How to Create a Chat-bot Using Django DRF
Conclusion
Serializers in Django Rest Framework are a fundamental component for handling the conversion of complex data types into formats suitable for rendering and parsing in the context of web APIs. They provide a flexible and powerful mechanism for serialization, deserialization, validation, and customization of the output format.
Whether you’re working with simple models or complex relationships between multiple models, DRF serializers offer a range of features to make the development of robust and efficient APIs a seamless process. Understanding how to use and customize serializers is key to harnessing the full potential of Django Rest Framework in building modern and scalable web applications.