How to Setup Pagination in DRF [Django Rest Framework]
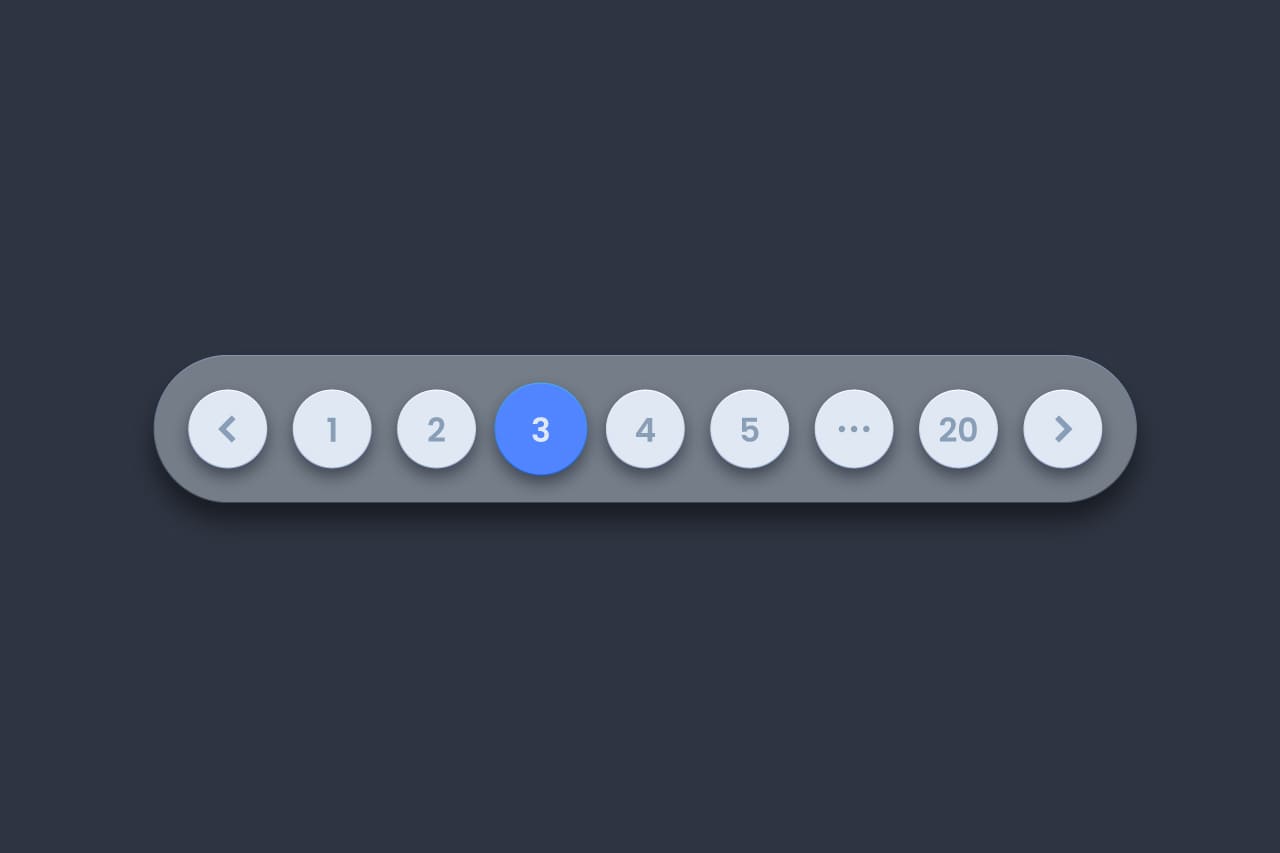
Pagination plays a crucial role in managing large datasets in web applications. When it comes to building APIs with Django Rest Framework (DRF), understanding and implementing pagination is essential for optimizing performance and improving the user experience.
In this blog post, we’ll explore the basics of pagination in DRF, discuss different pagination styles, and provide code examples to demonstrate how to implement pagination in Django Rest Framework-powered API.
Understanding Pagination in DRF:
Pagination in DRF involves breaking down a large set of data into smaller, more manageable chunks or pages. This not only enhances the efficiency of data retrieval but also prevents overwhelming the client with a massive amount of information in a single request. DRF supports various pagination styles, each with its characteristics.
To read more about setting up token authentication in Django Rest Framework, refer to our blog How to Set Up Token Authentication in Django Rest Framework
Pagination style in DRF:
1. PageNumberPagination:
This is the default pagination style in DRF, where the API response includes metadata such as the total number of pages, the current page number, and links to the next and previous pages.
# settings.py REST_FRAMEWORK = { 'DEFAULT_PAGINATION_CLASS': 'rest_framework.pagination.PageNumberPagination', 'PAGE_SIZE': 10, }
# views.py from rest_framework.pagination import PageNumberPagination from rest_framework.response import Response class MyListView(APIView): pagination_class = PageNumberPagination def get(self, request): queryset = MyModel.objects.all() page = self.paginate_queryset(queryset) if page is not None: serializer = MyModelSerializer(page, many=True) return self.get_paginated_response(serializer.data) serializer = MyModelSerializer(queryset, many=True) return Response(serializer.data)
2. LimitOffsetPagination:
This style allows the client to request a specific number of items (limit) and offset (starting point) within the overall dataset.
# settings.py REST_FRAMEWORK = { 'DEFAULT_PAGINATION_CLASS': 'rest_framework.pagination.LimitOffsetPagination', 'PAGE_SIZE': 10, }
# views.py from rest_framework.pagination import LimitOffsetPagination from rest_framework.response import Response class MyListView(APIView): pagination_class = LimitOffsetPagination def get(self, request): queryset = MyModel.objects.all() page = self.paginate_queryset(queryset) if page is not None: serializer = MyModelSerializer(page, many=True) return self.get_paginated_response(serializer.data) serializer = MyModelSerializer(queryset, many=True) return Response(serializer.data)
To read more about using the UPS Express API in Django DRF, refer to our blog How to Use the UPS Express API in Django DRF in 2024
Conclusion:
Implementing pagination in your DRF API is a crucial step in handling large datasets efficiently. The flexibility provided by DRF’s pagination styles allows you to tailor the pagination mechanism to suit the specific needs of your application. By following the examples provided in this blog post, you can seamlessly integrate pagination into your DRF project, ensuring optimal performance and a smoother user experience.