How to Implement Search Functionality Using Django Haystack & Whoosh in Your Django Project [2024]
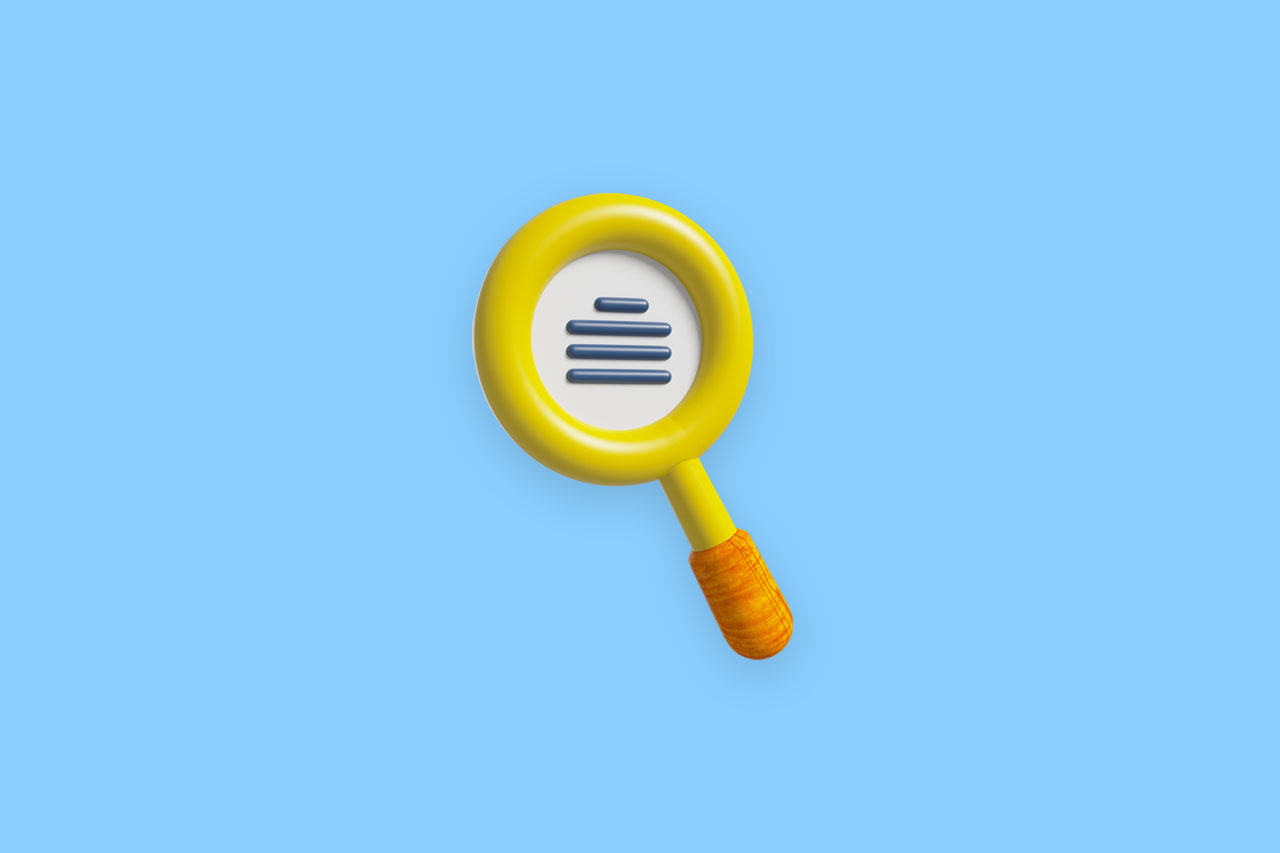
In the vast landscape of web development, search functionality plays a pivotal role in enhancing user experience. Whether you’re building a blog, an e-commerce platform, or any content-driven application, implementing efficient search capabilities can significantly improve navigation and user satisfaction. Fortunately, Django, a high-level Python web framework, provides powerful tools like Haystack and Whoosh to simplify the process of integrating search functionality into your projects.
In this blog post, we’ll explore how to incorporate search functionality using Django Haystack and Whoosh in a simple Django project.
By the end of this tutorial, you’ll have a solid understanding of how to leverage these tools to enable full-text search in your Django applications.
Setting Up the Environment
Before diving into the implementation, ensure you have Django, Haystack, and Whoosh installed in your Python environment. You can install them via pip:
pip install django django-haystack Whoosh
Once installed, let’s create a new Django project and app to work with:
django-admin startproject search_project cd search_project python manage.py startapp blog
Next, add ‘haystack’ and ‘blog’ to the INSTALLED_APPS list in your project’s settings.py file:
INSTALLED_APPS = [ ... 'haystack', 'blog', ]
Now, let’s configure Haystack to use Whoosh as the search engine. Add the following configurations to your settings.py file:
HAYSTACK_CONNECTIONS = { 'default': { 'ENGINE': 'haystack.backends.whoosh_backend.WhooshEngine', 'PATH': os.path.join(BASE_DIR, 'whoosh_index'), }, }
Don’t forget to import os at the top of your settings.py file:
import os
Defining Models
For this example, let’s consider a simple blog application with a Post model. Define your model in the models.py file of your blog app:
from django.db import models class Post(models.Model): title = models.CharField(max_length=100) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True) def __str__(self): return self.title
Creating Search Index
Now, we need to create a search index for our Post model. Create a file named search_indexes.py inside your blog app directory and define the index as follows:
from haystack import indexes from blog.models import Post class PostIndex(indexes.SearchIndex, indexes.Indexable): text = indexes.CharField(document=True, use_template=True) def get_model(self): return Post def index_queryset(self, using=None): return self.get_model().objects.all()
Configuring URLs
To enable search functionality, we need to configure URL patterns. Add the following line to the urls.py file in your project directory:
urlpatterns = [ ... path('search/', include('haystack.urls')), ]
Creating Templates
Create a directory named templates inside your blog app directory. Inside the templates directory, create a file named search/post_text.txt. This file will contain the template used for indexing the Post model. Add the following content to post_text.txt:
{{ object.title }} {{ object.content }}
Performing Search
Finally, let’s implement search functionality in our views. Open views.py in your blog app directory and define a view for searching posts:
from django.shortcuts import render from haystack.query import SearchQuerySet def search_posts(request): query = request.GET.get('q') results = SearchQuerySet().filter(content=query).load_all() return render(request, 'blog/search_results.html', {'results': results})
Creating Search Results Template
Create a file named search_results.html inside the templates/blog directory. This file will render the search results. Add the following content to search_results.html:
{% if results %}
<h2>Search Results:</h2>
<ul>
{% for result in results %}
<li><a href="{{ result.object.get_absolute_url }}">{{ result.object.title }}</a></li>
{% endfor %}
</ul>
{% else %}
<p>No results found.</p>
{% endif %}
Conclusion
In conclusion, the implementation of search functionality using Django Haystack and Whoosh in your Django project marks a significant enhancement in usability and discoverability. Through the steps outlined in this tutorial, you have acquired the essential knowledge to establish an efficient search system, covering the setup of the environment, definition of models, creation of search indexes, URL configuration, search execution, and presentation of search results.
These tools empower you to provide users with a seamless navigation experience and facilitate the retrieval of relevant content within your Django applications. As you continue to refine and expand your project, you are encouraged to explore additional customization options and advanced features to tailor the search functionality to meet specific requirements and further elevate user satisfaction. With the groundwork laid out in this tutorial, you are well-equipped to leverage Django Haystack and Whoosh effectively, fostering a dynamic and engaging user experience within your web applications. Harness these capabilities to their full potential and continue to innovate in your pursuit of building exceptional Django projects.