What are the Different Field Types in Django?
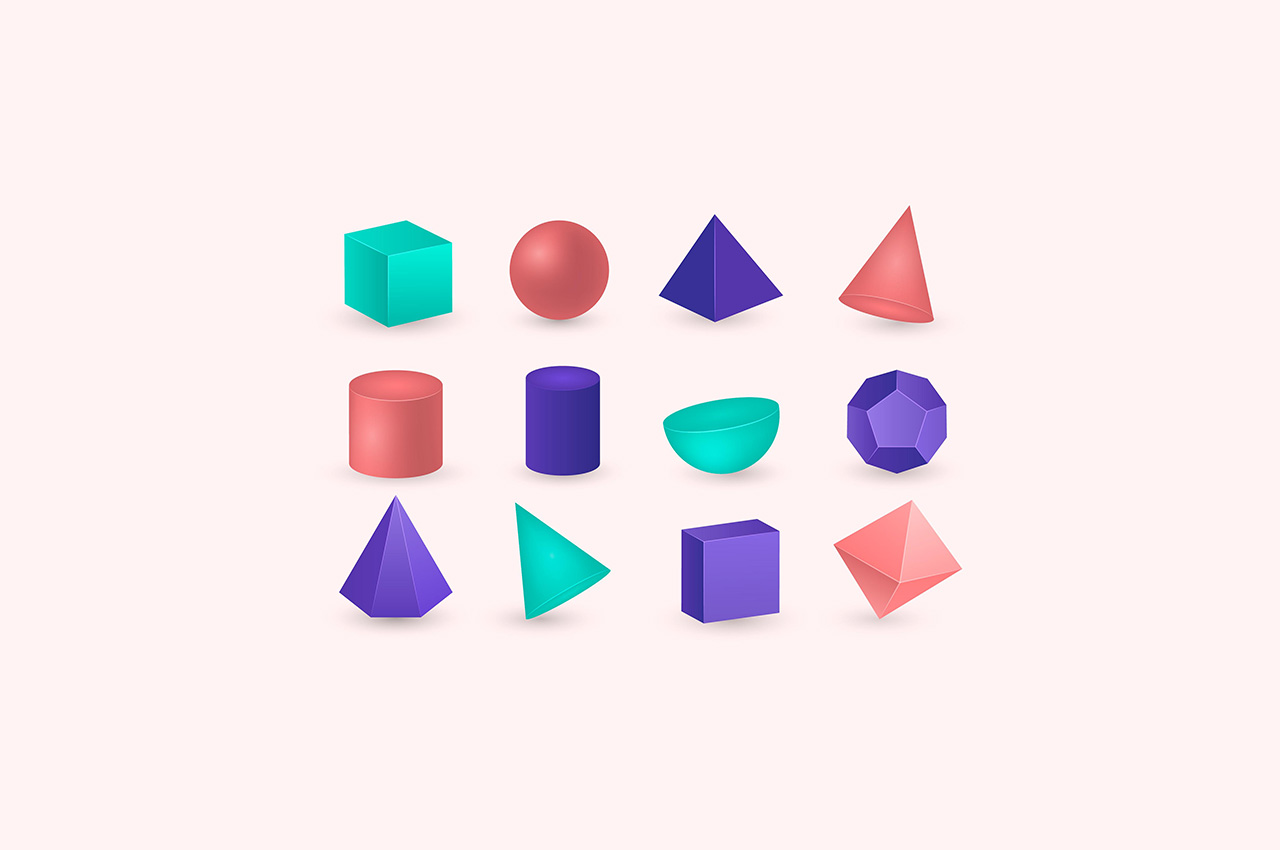
Django, a powerful web framework written in Python, provides a wide range of built-in field types to simplify database management. Understanding the different field types and their characteristics is essential for designing efficient and robust Django applications.
In this blog post, we will explore the various field types offered by Django and delve into their specific use cases and features.
1. CharField:
The CharField is a fundamental field type used to store string data. It allows for specifying a maximum length, making it suitable for storing short to medium-sized strings such as names, titles, or descriptions. This field type is indexed by default and supports various options such as default values, blank values, and unique constraints.
Example usage:
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.CharField(max_length=50)
description = models.TextField()
In the above example, the `title` and `author` fields are defined as CharField to store the book’s title and author’s name, respectively. The `max_length` parameter is set to limit the length of the strings.
2. TextField:
The TextField is similar to the CharField but is designed to store larger amounts of text. It does not have a predefined length limit, making it suitable for storing extensive content such as blog posts, articles, or user comments. This field type is useful when you need to store text with no predetermined size restrictions.
Example usage:
class BlogPost(models.Model):
title = models.CharField(max_length=100)
content = models.TextField()
In the above example, the `content` field is defined as TextField to store the extensive content of a blog post.
3. IntegerField:
The IntegerField is used to store integer values. It provides options to define minimum and maximum values, as well as default and nullability settings. This field type ensures that only valid integers are stored in the database.
Example usage:
class Product(models.Model):
name = models.CharField(max_length=50)
price = models.IntegerField()
In the above example, the `price` field is defined as an IntegerField to store the price of a product as an integer value.
4. FloatField:
The FloatField is used to store floating-point numbers. It allows specifying the number of decimal places and supports all the options provided by the IntegerField. This field type is suitable for storing values that require decimal precision.
Example usage:
class Rating(models.Model):
value = models.FloatField()
In the above example, the value field is defined as FloatField to store a rating value with decimal precision.
5. BooleanField:
The BooleanField is used to store boolean values, representing either true or false. It is commonly used to model yes/no or on/off choices in a database. This field type is helpful when you need to represent binary choices.
Example usage:
class Task(models.Model):
name = models.CharField(max_length=50)
is_completed = models.BooleanField(default=False)
In the above example, the `is_completed` field is defined as BooleanField to indicate whether a task is completed or not.
6. DateField and DateTimeField:
DateField and DateTimeField are used to store dates and date-time values, respectively. DateField stores only the date, while DateTimeField stores both the date and time information. These field types offer various options for defining default values, auto-populating values, and enforcing specific date ranges.
Example usage:
class Event(models.Model):
name = models.CharField(max_length=100)
date = models.DateField()
start_time = models.DateTimeField()
In the above example, the `date` field is defined as DateField to store the date of an event, and the `start_time` field is defined as DateTimeField to store the date and time when the event starts.
7. EmailField:
The EmailField is designed specifically for storing email addresses. It ensures that the entered value matches the format of a valid email address. This field type validates the input and helps maintain consistency in storing email addresses.
Example usage:
class UserProfile(models.Model):
email = models.EmailField(unique=True)
In the above example, the `email` field is defined as EmailField to store the email address of a user. The `unique=True` option ensures that each email address is unique within the database.
8. ForeignKey and ManyToManyField:
ForeignKey and ManyToManyField are special field types used to establish relationships between different database models. ForeignKey represents a one-to-many relationship, where one model has a reference to another model. ManyToManyField represents a many-to-many relationship, where multiple models are connected to multiple other models.
Example usage (ForeignKey):
class Author(models.Model):
name = models.CharField(max_length=50)
class Book(models.Model):
title = models.CharField(max_length=100)
author = models.ForeignKey(Author, on_delete=models.CASCADE)
In the above example, the `Book` model has a ForeignKey field named `author` that references the `Author` model. This establishes a one-to-many relationship where an author can have multiple books.
Example usage (ManyToManyField):
class Tag(models.Model):
name = models.CharField(max_length=50)
class BlogPost(models.Model):
title = models.CharField(max_length=100)
tags = models.ManyToManyField(Tag)
In the above example, the `BlogPost` model has a ManyToManyField named `tags` that references the `Tag` model. This establishes a many-to-many relationship where a blog post can have multiple tags, and a tag can be associated with multiple blog posts.
To read more about the key features of Django model forms, refer to our blog Key Features of Django Model Forms in 2023
Conclusion:
Understanding the various field types offered by Django is crucial for developing robust and efficient web applications. By choosing the appropriate field types, developers can ensure data integrity, enforce constraints, and optimize database interactions. This blog post provided an overview of some of the commonly used field types in Django, including CharField, TextField, IntegerField, FloatField, BooleanField, DateField, DateTimeField, EmailField, and the relationship field types ForeignKey and ManyToManyField. By incorporating these field types effectively, you can build dynamic and feature-rich Django applications.