How to Enable Internationalization & Localization in Django [2024]
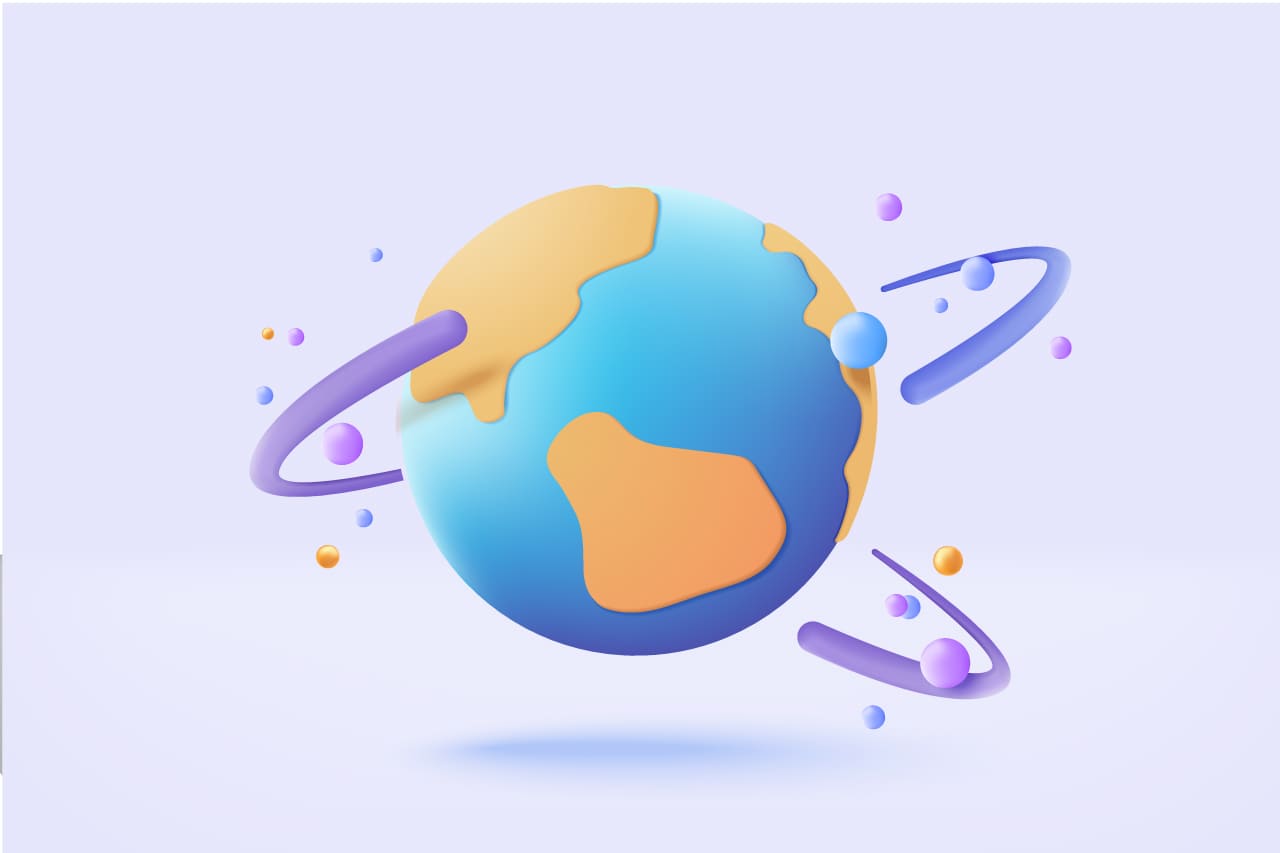
In the ever-expanding digital landscape, the ability to reach a global audience is paramount. For web developers using Django, the popular Python web framework, internationalization (i18n) and localization (l10n) features play a crucial role in making applications accessible to users around the world.
In this blog post, we’ll delve into the significance of internationalization and localization in Django and explore how these features empower developers to support multiple languages seamlessly.
Understanding Internationalization and Localization:
Internationalization refers to the process of designing and preparing software to be adaptable to different languages and regions without modification. It involves isolating translatable content, such as strings and date formats, from the codebase, making it easier to cater to diverse linguistic and cultural preferences.
Localization, on the other hand, is the process of adapting an internationalized application to a specific locale or language. It includes translating text, formatting numbers and dates according to regional conventions, and handling other locale-specific nuances.
Enabling Internationalization in Django:
Django simplifies the internationalization process through its built-in support for i18n. To get started, developers need to follow key steps:
1. Activate Internationalization
- Set the USE_I18N setting to True in the project’s settings file.
- Add ‘django.middleware.locale.LocaleMiddleware’ to the MIDDLEWARE setting.
- The USE_L10N setting in Django controls whether to enable or disable the localization of numbers, dates, and times in your application
# settings.py # Activate internationalization USE_I18N = True USE_L10N = True MIDDLEWARE = [ # ... 'django.middleware.locale.LocaleMiddleware', # ... ]
2. Configure Supported Languages and Translation Paths:
- Use the LANGUAGE_CODE setting for the default language
- The LANGUAGES setting for the supported languages. Each language should be represented as a tuple containing a language code and its human-readable name
- Specify the directory where Django will look for translation files using the LOCALE_PATHS setting. This is crucial for the make message management command to generate translation templates.
# Set the default language for your application LANGUAGE_CODE = 'en-us' # Specify the languages your application supports LANGUAGES = [ ('en', _('English')), ('es', _('Spanish')), ('fr', _('French')), # Add more languages as needed ] # Set the directory where Django will look for translations LOCALE_PATHS = [ os.path.join(BASE_DIR, 'locale'), # Adjust the path based on your project structure ]
3. Marking Translatable Strings:
- Both gettext and `gettext_lazy` are used to mark strings for translation.
- `gettext` is suitable for immediate translations in Python code. When applied to a string, it tells Django’s translation utilities to extract and include that string in the translation files.
from django.utils.translation import gettext as _ # Marking a string for translation welcome_message = _("Welcome to our website!")
- `gettext_lazy` is used in situations where deferred translation is preferred. It is primarily used for marking strings in lazy translations. Lazy translations are deferred until the actual translation is needed, which can be beneficial in certain situations, such as when working with Django model fields or queryset lookups.
from django.utils.translation import gettext_lazy as _ # Using gettext_lazy with a model field class MyModel(models.Model): name = models.CharField(max_length=255, verbose_name=_("Name"))
4. Using {% trans %} in Django HTML Templates:
- When it comes to rendering translated strings in Django templates, the {% trans %} template tag is your go-to tool. Here’s an example illustrating how to use it.
<!-- template.html -->
<!DOCTYPE html>
<html lang="{% get_current_language %}">
<head>
<meta charset="UTF-8">
<title>{% trans "My Multilingual Website" %}</title>
</head>
<body>
<h1>{% trans "Welcome to our site!" %}</h1>
<p>{% trans "This is a sample paragraph in multiple languages." %}</p>
</body>
</html>
5. Create Language Switching URLs:
- In your urls.py file, include a URL pattern for language switching. This pattern will map to the set_language view provided by Django.
# urls.py from django.urls import path from django.views.i18n import set_language urlpatterns = [ # ... other URL patterns ... path('set-language/', set_language, name='set_language'), # ... other URL patterns ... ]
6. Update the Template to Include Language Switching:
- In your template(s), include a way for users to select their preferred language. This could be in the form of a dropdown menu, buttons, or any other user interface element.
7. Create Translation Files:
- Run the makemessages management command to create a .po file containing translatable strings.
# Replace 'es' with the target language code python manage.py makemessages -l es # Create .po file for whole languages given in settings.py file python manage.py makemessages –all
8. Edit the .po File:
- Open the generated .po file for the desired language in a text editor. It will be located in the locale directory specified in your LOCALE_PATHS setting. For example, if you generated Spanish translations, you might have a file like locale/es/LC_MESSAGES/django.po.
9. Add Translations:
- Locate the msgid entries and add the corresponding translations in the msgstr fields.
msgid "Hello, World!" msgstr "¡Hola, Mundo!"
10. Compile Translations:
- After adding translations to the .po file, run the following command to compile the translations into a binary .mo file, which Django can use for efficient language-switching
python manage.py compilemessages
11. Test Translations:
- Restart your Django development server and set the language to the one you added translations for. You should see the translated strings in your application.
Conclusion:
In a world that thrives on diversity, the ability to offer applications in multiple languages is a strategic advantage for web developers. Django’s robust internationalization and localization support empower developers to create inclusive and globally accessible applications. By following best practices and leveraging Django’s built-in features, developers can navigate the intricacies of language support with ease, ensuring that their applications resonate with users from various linguistic backgrounds. Embracing internationalization and localization is not just a technical necessity but a commitment to making the digital realm more inclusive and welcoming for all.