How to Retrieve Data From Django ORM Queries [Ultimate Guide]
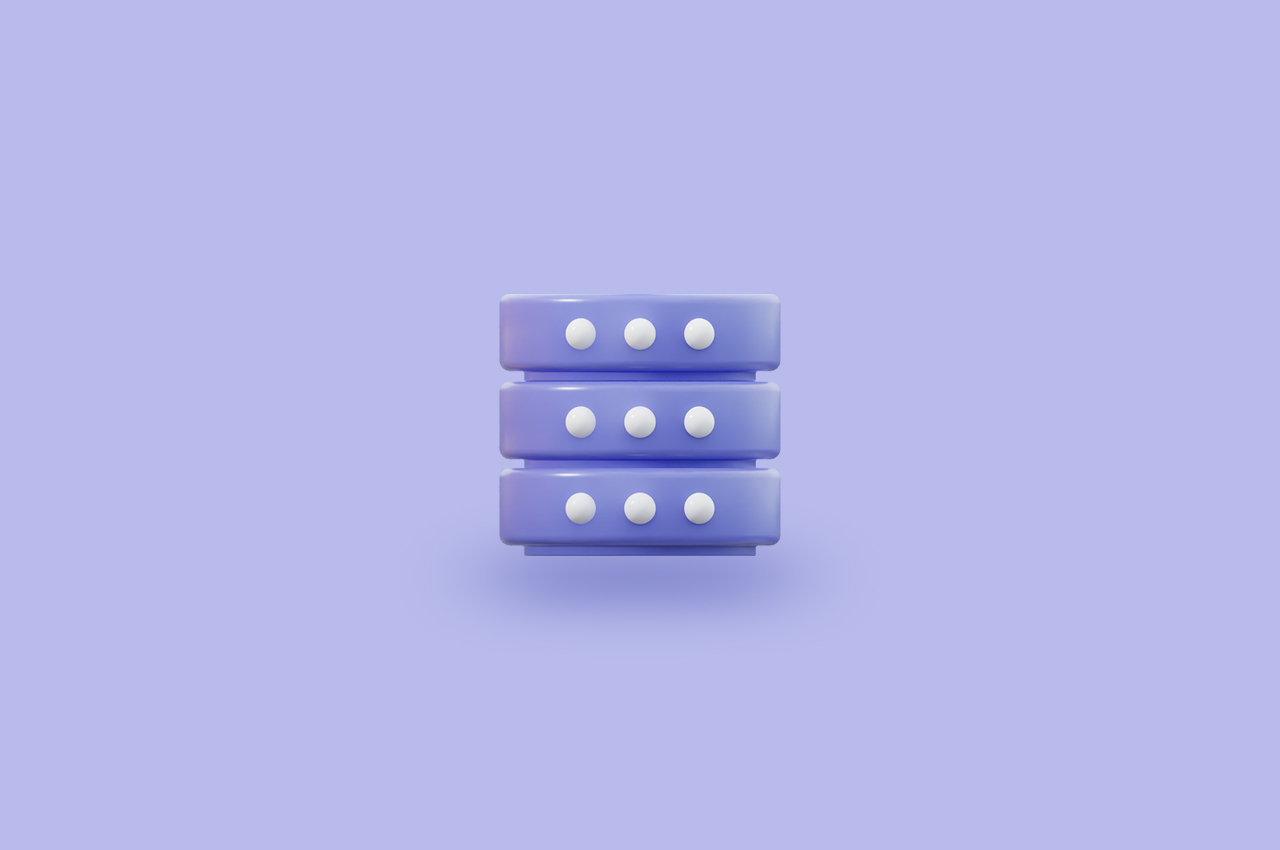
Django, the popular Python web framework, provides developers with a powerful tool called QuerySets, which simplifies database interactions and empowers efficient data retrieval. QuerySets allow you to fetch, filter, and manipulate data effortlessly. In this blog post, we will explore the versatility of Django QuerySets, understand their functionalities, and showcase practical examples of how they can be used effectively.
Sample Model:
Before diving into the examples, let’s create a sample model to work with throughout this blog. Consider a simple e-commerce application where we have a Product model that represents various products available for sale. Here’s an example of the Product model:
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
category = models.CharField(max_length=100)
price = models.DecimalField(max_digits=8, decimal_places=2)
def __str__(self):
return self.name
Understanding QuerySets:
A QuerySet in Django represents a collection of objects from a specific model obtained through querying the database. QuerySets offer a rich set of operations and methods to manipulate and retrieve data efficiently. Let’s delve into the essential features and showcase practical examples of each.
Retrieving Objects:
The primary purpose of QuerySets is to retrieve objects from the database. Django provides various methods to achieve this, such as all(), get(), filter(), first(), and exists(). Let’s look at some examples:
from myapp.models import Product
# Retrieve all products
all_products = Product.objects.all()
print(all_products) # Output: <QuerySet [<Product: Product 1>, <Product: Product 2>, ...]>
# Retrieve a specific product by its primary key
product = Product.objects.get(pk=1)
print(product) # Output: <Product: Product 1>
# Retrieve products that match specific criteria
filtered_products = Product.objects.filter(category='electronics')
print(filtered_products) # Output: <QuerySet [<Product: Product 1>, <Product: Product 3>, ...]>
# Retrieve the first product in the queryset
first_product = Product.objects.first()
print(first_product) # Output: <Product: Product 1>
# Check if any products exist in the queryset
products_exist = Product.objects.exists()
print(products_exist) # Output: True
Chaining Methods:
QuerySets allow you to chain methods, constructing complex queries with ease. By chaining methods, you can refine the results further. Here’s an example:
# Retrieve all electronics products with a price greater than $500
products = Product.objects.filter(category='electronics').filter(price__gt=500)
print(products) # Output: <QuerySet [<Product: Product 3>, <Product: Product 4>, ...]>
Lazy Evaluation:
QuerySets offer lazy evaluation, which optimizes performance by deferring database operations until necessary. It reduces the number of queries executed. Let’s see an example:
# QuerySet is created but not executed immediately
products = Product.objects.filter(category='electronics')
# The query is executed when the data is accessed or iterated
for product in products:
print(product.name)
# Output: Product 1, Product 3, ...
Aggregation and Annotation:
QuerySets provide aggregation methods like count(), sum(), average(), and annotate(). These methods allow you to perform mathematical operations and retrieve aggregated data. Here’s an example:
from django.db.models import Count, Avg
# Count the number of products in each category
category_counts = Product.objects.values('category').annotate(count=Count('id'))
print(category_counts)
# Output: <QuerySet [{'category': 'electronics', 'count': 5}, {'category': 'clothing', 'count': 3}, ...]>
# Calculate the average price of all products
average_price = Product.objects.aggregate(avg_price=Avg('price'))
print(average_price) # Output: {'avg_price': 450.0}
Ordering Results:
Django QuerySets enable sorting results based on specific fields using the order_by() method. You can specify fields and even apply descending order. Here’s an example:
# Retrieve products ordered by price in ascending order
products = Product.objects.order_by('price')
print(products) # Output: <QuerySet [<Product: Product 2>, <Product: Product 1>, ...]>
# Retrieve products ordered by price in descending order
products = Product.objects.order_by('-price')
print(products) # Output: <QuerySet [<Product: Product 3>, <Product: Product 4>, ...]>
Relationship Traversal:
QuerySets support traversing relationships between models efficiently. You can access related objects using the related_name attribute or the double underscore (__) notation. Let’s see an example:
from myapp.models import Order
# Retrieve all orders related to a specific customer
customer_orders = Order.objects.filter(customer__name='John Doe')
print(customer_orders) # Output: <QuerySet [<Order: Order 1>, <Order: Order 2>, ...]>
Additional Methods:
Apart from the methods mentioned above, Django QuerySets provide several other useful methods for data retrieval and manipulation. Here are a few more worth exploring:
create(): Creates a new object and saves it to the database. Here’s an example:
new_product = Product.objects.create(name='New Product', category='electronics', price=999.99)
print(new_product) # Output: <Product: New Product>
exclude(): Retrieves objects that do not match the specified criteria. Here’s an example:
non_electronics_products = Product.objects.exclude(category='electronics')
print(non_electronics_products) # Output: <QuerySet [<Product: Product 2>, <Product: Product 4>, ...]>
values(): Retrieves a subset of fields from the objects. Here’s an example:
product_names = Product.objects.values('name')
print(product_names) # Output: <QuerySet [{'name': 'Product 1'}, {'name': 'Product 2'}, ...]>
distinct(): Retrieves unique objects, eliminating duplicates. Here’s an example:
unique_categories = Product.objects.values('category').distinct()
print(unique_categories) # Output: <QuerySet [{'category': 'electronics'}, {'category': 'clothing'}, ...]>
Conclusion:
Django QuerySets provide a powerful and flexible way to interact with the database and retrieve data. By understanding their functionalities and leveraging their features effectively, you can simplify complex queries and optimize data retrieval in your Django applications. Experiment with QuerySets, explore the available methods, and unlock the full potential of data manipulation in Django.
To read more about configuring HTMX in Django project refer to our blog How to Configure HTMX in Django Project: The Complete Guide