How to Track History in Django using django-simple-history in 2024
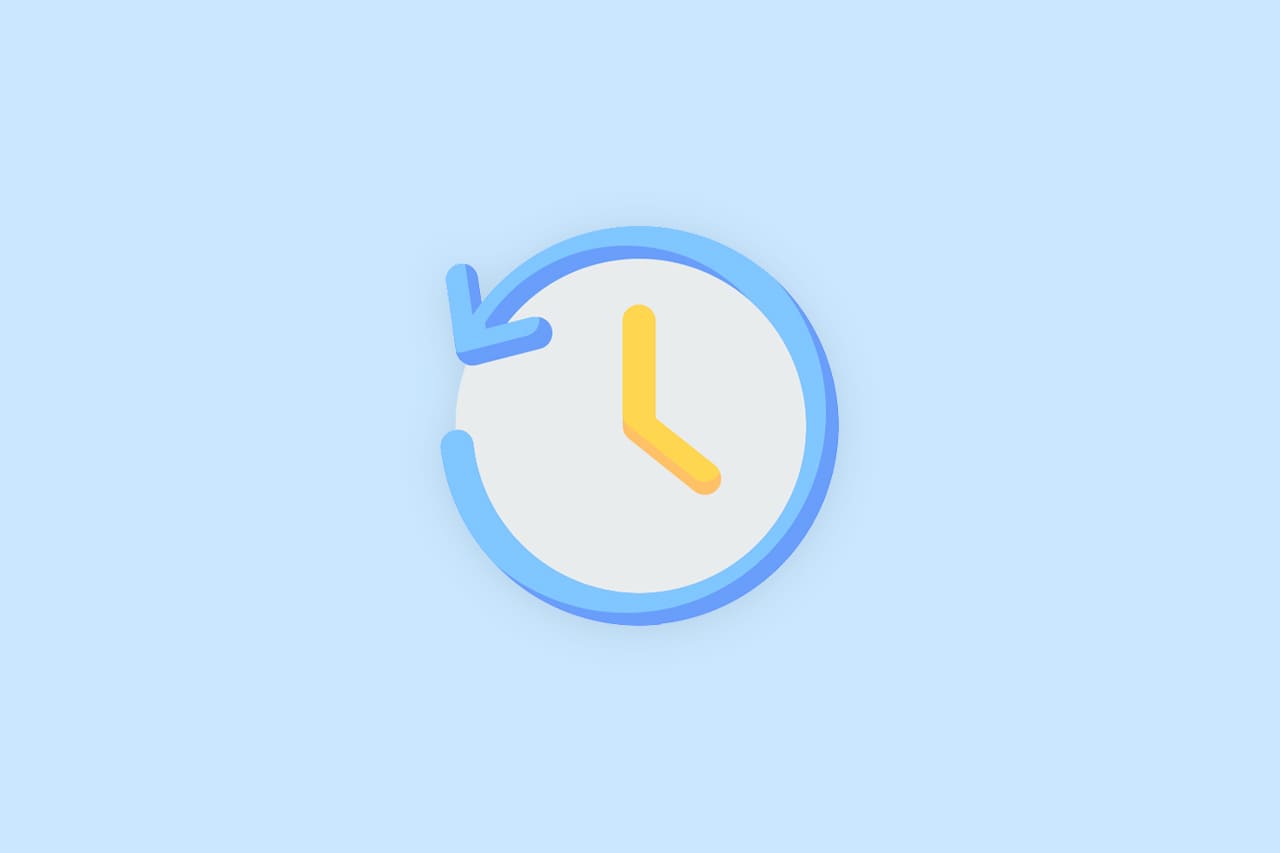
What is History Tracking?
This means you can always go back in time and see how your data looked at any point in the past. It’s like having a record of all the edits, like a logbook, showing who made each change and when it happened. This is incredibly useful for auditing, troubleshooting, or simply understanding the evolution of your data over time.
What is django-simple-history
Django-simple-history is a Django app that provides history tracking functionality for your models. It allows you to keep track of changes made to your data over time by automatically recording each modification, addition, or deletion of model instances. This means you can easily see the history of changes made to your database records, including who made the changes and when they occurred.
How to install django-simple-history
This app is compatible with the following combinations of Python and Django versions.
Django | Python |
3.2 | 3.8,3.9,3.10 |
4.2 | 3.8,3.9,3.10,3.11,3.12,3.13-dev |
5.0 | 3.10,3.11,3.12,3.13-dev |
main | 3.10,3.11,3.12,3.13-dev |
To read more about setting up a Django Project, refer to our blog How to Set Up a Django Project in 10 Steps
Setup procedure
1. Install django-simple-history:
You can install it via pip:
pip install django-simple-history
2. Add it to your INSTALLED_APPS and MIDDLEWARE
In your Django project’s settings.py, add ‘simple_history’ to the INSTALLED_APPS list:
INSTALLED_APPS = [ # ... 'simple_history', ]
To automatically populate the history user and track who made each change, you can include the HistoryRequestMiddleware in your Django settings.
MIDDLEWARE = [ # ... 'simple_history.middleware.HistoryRequestMiddleware', ]
3. Register models for tracking:
In the models.py of the app where you want to track changes, import `SimpleHistory` and use it as a mixin class for your models.
from django.db import models from simple_history.models import HistoricalRecords class YourModel(models.Model): # Your model fields here history = HistoricalRecords()
4. Run migrations
After adding history tracking to your models, run:
python manage.py makemigrations python manage.py migrate
5. Accessing historical records:
Once you’ve set up history tracking, you can access historical records using the `history` attribute on your model instances.
# Retrieve the history of a specific instance instance.history.all() # Access the historical fields of a specific version history_instance = instance.history.get(pk=history_id) history_instance.field_name
6. Admin integration:
django-simple-history provides an admin integration to view historical changes directly in the Django admin interface. To enable it, register your models with `HistoricalRecordsAdmin.`
from django.contrib import admin from simple_history.admin import SimpleHistoryAdmin class YourModelAdmin(SimpleHistoryAdmin): pass admin.site.register(YourModel, YourModelAdmin)
7. For existing projects:
For projects already in progress, you can utilize the populate command to generate an initial change for existing model instances:
$ python manage.py populate_history --auto
By default, history entries are inserted in batches of 200. If necessary for larger tables, you can adjust this batch size using the –batchsize option, such as –batchsize 500.
To read more about building a real-time notification system with Django-notifications-hq, refer to our blog How to Build a Real-Time Notification System With Django-notifications-hq
Conclusion
In conclusion, Django Simple History offers a seamless solution for tracking changes to your Django models over time. By incorporating HistoricalRecords into your models, you can effortlessly record and access the history of modifications made to your data. This functionality provides transparency, enhances data integrity, and facilitates auditing and troubleshooting processes within your Django projects.
Furthermore, for existing projects, the populate command allows for the generation of initial change records for preexisting model instances, offering a smooth integration process. With its flexibility and ease of use, Django Simple History proves to be a valuable tool for developers seeking comprehensive history tracking capabilities in their Django applications.