How to Create Custom Model Fields in Django [2024]
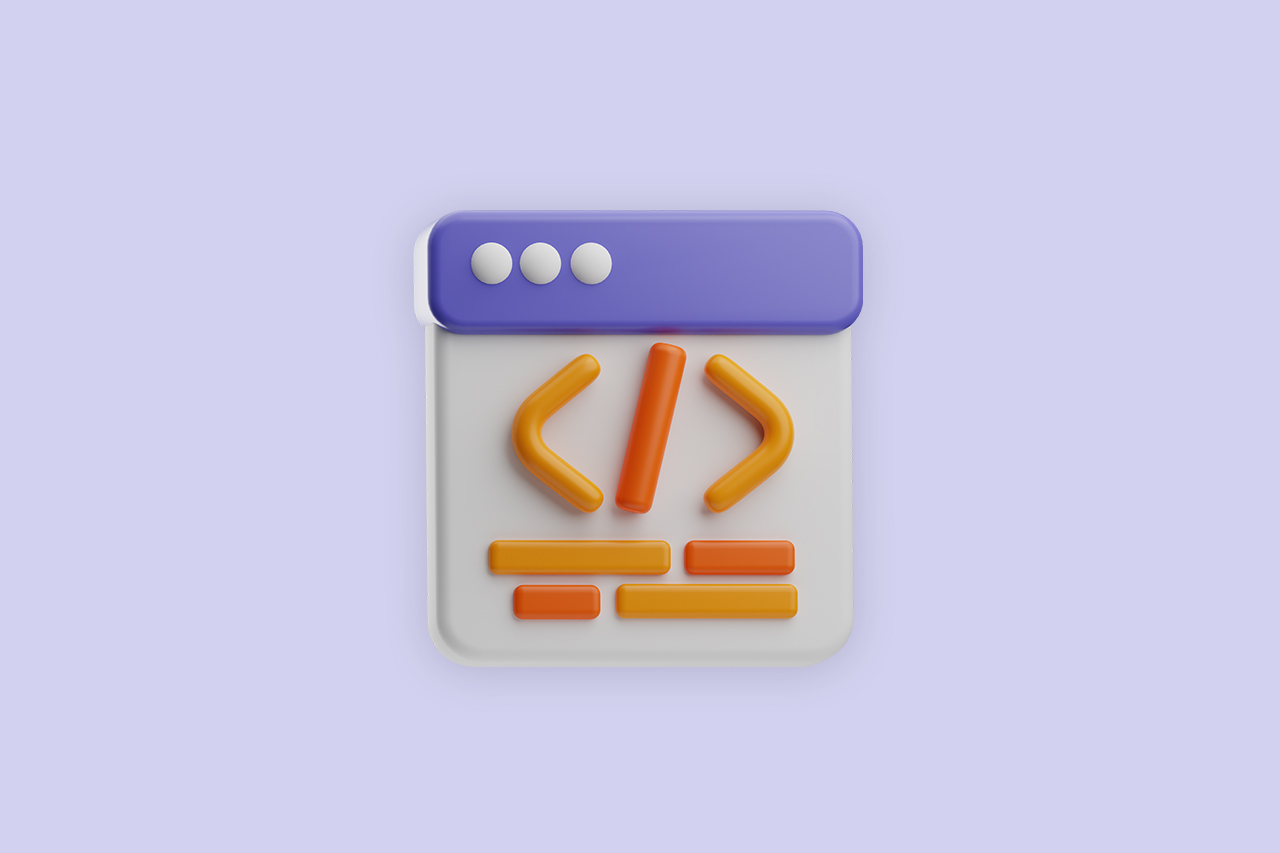
Django’s flexibility and extensibility make it a powerful web framework, allowing developers to create custom solutions tailored to their specific needs. In this blog post, we’ll explore the process of creating a new Django model field. By developing a custom model field, you can enhance your Django projects with specialized data types, extending the framework’s capabilities.
Understanding Django Model Fields:
Django model fields play a pivotal role in defining the structure of a database schema within a Django application. They serve as the bridge between the Python objects in your application and the underlying relational database. By encapsulating the characteristics of various data types, model fields allow developers to seamlessly interact with and manipulate data stored in the database.
Django provides a rich set of built-in model fields that cover a wide range of data types, including integers, text, dates, and relationships. Each field is designed to represent a specific type of data and is equipped with various options to customize its behavior. For instance, a CharField might represent a short text string, while an IntegerField is suitable for storing whole numbers.
These fields not only define the type of data but also enforce constraints, such as maximum length, choices, and nullability, ensuring the integrity and consistency of the data stored in the database.
To read more about building a custom user model in Django, refer to our blog How to Build a Custom User Model in Django
Introduction to the Need for a Custom Model Field:
While Django’s built-in model fields are versatile and cover a broad spectrum of use cases, there are situations where a custom model field becomes a necessity. The need for customization arises when dealing with data types or structures not readily addressed by Django’s default set of fields.
Here are some scenarios where a custom model field may be required:
- Specialized Data Types: Handling data types that are not standard in relational databases, such as geographic coordinates, encrypted data, or custom serialized objects.
- Complex Validation Logic: Implementing complex validation logic specific to a certain type of data. For example, validating a custom identifier format or implementing business-specific rules.
In the upcoming sections, we will explore the step-by-step process of creating a custom model field in Django, allowing developers to address these specific needs and extend the framework’s capabilities.
1. Setting Up Your Django Project :
- Creating a new Django project or using an existing one for implementing the custom model field.
- Preparing the project structure and ensuring proper virtual environment setup. (refer to our blog How to Set Up a Django Project in 10 Steps)
2. Creating the Custom Model Field :
a. Defining the Field Class:
- Create a new Python class that extends django.db.models.Field in the customfields.py file within your project folder.
- Implementing the __init__ method to set field-specific attributes.
# customfields.py from django.db import models class CustomPhoneNumberField(models.Field): def __init__(self, *args, **kwargs): kwargs['max_length'] = 15 super().__init__(*args, **kwargs)
b. Implementing deconstruct Method:
- Ensuring the field can be serialized for migrations.
def deconstruct(self): name, path, args, kwargs = super().deconstruct() return name, path, args, kwargs
c. Overriding db_type Method:
- Specifying the database column type for the custom field.
def db_type(self, connection): return 'varchar(15)'
3. Integrating the Custom Model Field:
- Importing and using the custom field in your models.
# models.py from django.db import models from .customfields import CustomPhoneNumberField class Contact(models.Model): name = models.CharField(max_length=100) phone_number = CustomPhoneNumberField()
4. Handling Serialization and Deserialization:
- Implementing methods to convert the custom field value between Python and database representations in the custom field class.
def from_db_value(self, value, expression, connection): return self.to_python(value) def to_python(self, value): if value is None: return value return str(value)
Conclusion:
In conclusion, creating custom model fields in Django adds a layer of flexibility and adaptability to your projects, enabling you to tailor your database schema to unique data types and validation requirements. While Django’s built-in model fields cover a broad range of scenarios, there are situations where a more specialized approach is necessary. This blog post has guided you through the process of developing a custom model field, using a practical example of a CustomPhoneNumberField.