How to Implement Captcha in Django in 2023?
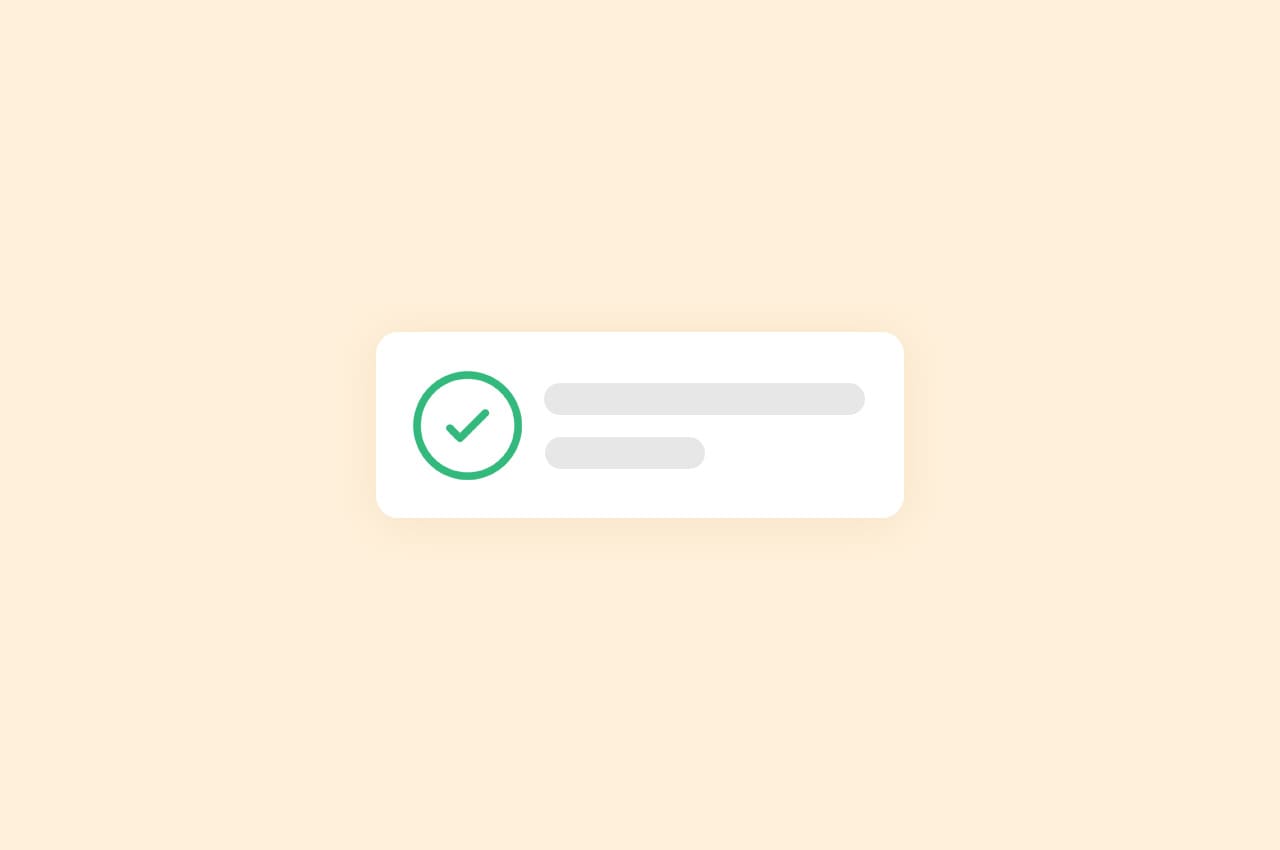
Ever been annoyed by spammy forms and robot-like behavior on websites? That’s where CAPTCHA comes in. We’re here to break down how to use CAPTCHA with Django, the friendly Python web framework.
Imagine a puzzle that only humans can solve. That’s CAPTCHA—your website’s bouncer against sneaky bots. We’ll show you how to set up your Django project, add CAPTCHA to your forms, and even style it to match your site. No tech jargon, just easy steps to safeguard your site from unwanted automated mischief. Join us to make your online world safer with CAPTCHA!
Step 1: Setting up Your Django Project
Before diving into CAPTCHA implementation, ensure you have a Django project up and running. If not, follow these steps:
Install Django: If you haven’t already, install Django using the following command:
pip install django
Create a Project: Create a new Django project using the following command, replacing “projectname” with your desired project name:
django-admin startproject projectname
Navigate to Project Directory: Move to your project’s directory using:
cd projectname
Create a Django App: Now, create a new app within your project. An app is a modular component of your project:
python manage.py startapp yourappname
Include the App: Add your app to the project’s settings. Open settings.py within the projectname folder, locate the INSTALLED_APPS list, and add ‘yourappname’ to the list.
Now you have the basic structure of your Django project set up. You’re ready to move on to adding CAPTCHA functionality!
Step 2: Selecting a CAPTCHA Service
To effectively defend your forms from spam bots, you need a reliable CAPTCHA service. There are various options available, but we’ll use Google reCAPTCHA for this guide. Here’s how to get started:
Sign Up for reCAPTCHA:
Head to the reCAPTCHA website and sign in with your Google account. Once logged in, click the “+ button” to register a new site. Fill in the necessary details, including your domain.
Get Your Keys:
After registration, you’ll receive two keys: Site Key and Secret Key. These keys are vital for integrating reCAPTCHA into your Django app. Keep them secure; you’ll need them in the upcoming steps.
Choose reCAPTCHA Version:
reCAPTCHA offers both v2 and v3. For v2, users solve image-based puzzles, while v3 operates in the background without user interaction. Choose the version that best fits your needs.
Adding Keys to Your Django Settings:
Open your Django project’s settings.py file and locate the section where you define your project’s settings. Add the following lines, replacing ‘your-site-key’ and ‘your-secret-key’ with the actual keys you received from reCAPTCHA:
RECAPTCHA_SITE_KEY = 'your-site-key'
RECAPTCHA_SECRET_KEY = 'your-secret-key'
You’ve now selected reCAPTCHA as your CAPTCHA service and obtained the necessary keys. In the next step, we’ll incorporate the reCAPTCHA widget into your forms to effectively prevent automated spam.
Step 3: Integrating reCAPTCHA with Your Forms
Now that you have your reCAPTCHA keys, it’s time to integrate the reCAPTCHA widget into your Django forms to enhance security against spam bots. Here’s how:
Update Your Form:
Open the Django form you want to protect from bots. Import the necessary modules at the beginning of your forms.py file:
from django import forms
from django.forms import ValidationError
from django.conf import settings
import requests
Add reCAPTCHA Field:
Inside your form class, add a reCAPTCHA field. This field will render the reCAPTCHA widget in your form:
class YourFormName(forms.Form):
# Your existing fields here
recaptcha = forms.CharField(widget=forms.HiddenInput())
Update Your Template:
In the template where you render your form, add the reCAPTCHA widget using the provided site key. Place the following code where you want the reCAPTCHA widget to appear:
<div class="g-recaptcha" data-sitekey="{{ settings.RECAPTCHA_SITE_KEY }}"></div>
Process reCAPTCHA Verification:
In your form’s view function, validate the reCAPTCHA response before processing the form data. Add the following code to the beginning of your view function:
def your_view_function(request):
if request.method == 'POST':
recaptcha_response = request.POST.get('g-recaptcha-response')
payload = {
'secret': settings.RECAPTCHA_SECRET_KEY,
'response': recaptcha_response
}
response = requests.post('https://www.google.com/recaptcha/api/siteverify', data=payload)
result = response.json()
if not result['success']:
# Handle failed reCAPTCHA verification here
pass
else:
# Process your form data
pass
else:
# Handle GET request or render form
pass
You’ve successfully integrated reCAPTCHA into your Django form, strengthening your website’s defenses against spam bots. In the next step, we’ll tackle how to style the reCAPTCHA widget and provide user-friendly feedback.
Step 4: Styling the reCAPTCHA Widget and User Feedback
Enhance the user experience by customizing the appearance of the reCAPTCHA widget and providing clear feedback messages. This ensures your users can easily interact with the CAPTCHA while understanding its purpose.
Customize Widget Appearance:
You can style the reCAPTCHA widget to match your website’s design. Adjust the widget’s size, theme, and other attributes by modifying the HTML code in your template:
<div class="g-recaptcha" data-sitekey="{{ settings.RECAPTCHA_SITE_KEY }}" data-theme="light" data-size="normal"></div>
Provide Feedback Messages:
To keep users informed about CAPTCHA’s purpose and potential errors, create helpful messages in your form template. For example, near the reCAPTCHA widget, you can display a message like:
<p>Please complete the CAPTCHA to verify that you're not a robot.</p>
In your view function, if the reCAPTCHA verification fails, send an error message back to the template:
if not result['success']:
error_message = "CAPTCHA verification failed. Please try again."
return render(request, 'your_template.html', {'error_message': error_message})
In your view function, if the reCAPTCHA verification fails, send an error message back to the template:
if not result['success']:
error_message = "CAPTCHA verification failed. Please try again."
return render(request, 'your_template.html', {'error_message': error_message})
Then, include this error message in your template to guide users:
{% if error_message %}
<p class="error">{{ error_message }}</p>
{% endif %}
Test and Refine:
Before deploying your CAPTCHA-enabled form, thoroughly test it. Verify that the styling aligns with your website’s design, and ensure that the feedback messages are clear and easy to understand. If needed, adjust the styling or messages for better usability.
By personalizing the reCAPTCHA widget’s appearance and providing user-friendly feedback, you’ll create a seamless experience for your website visitors while safeguarding against unwanted bot submissions.
Step 5: Wrapping Up and Further Security Tips
Congratulations! You’ve successfully implemented CAPTCHA protection in your Django forms, making your website more secure against automated spam and malicious bots. Here’s a summary of what you’ve accomplished and a few additional tips to consider:
Summary of Implementation:
- Setting up Your Project: You started by creating a new Django project and app.
- Selecting reCAPTCHA: You chose Google reCAPTCHA as your CAPTCHA service and obtained the required Site Key and Secret Key.
- Integrating reCAPTCHA: You integrated the reCAPTCHA widget into your Django forms and verified user responses using the keys.
- Styling and Feedback: You customized the reCAPTCHA widget’s appearance and provided clear feedback messages for a user-friendly experience.
- Further Security Considerations: You learned how to enhance security while maintaining usability.
Additional Security Tips:
- Implement Rate Limiting: To prevent attackers from overwhelming your server with multiple requests, implement rate limiting for CAPTCHA verification requests.
- Use HTTPS: Ensure your website uses HTTPS to encrypt data transmission between users and your server, protecting CAPTCHA data as well.
- Regularly Update Dependencies: Keep your Django project and CAPTCHA libraries up to date to benefit from security patches and improvements.
- Monitor for Abuse: Regularly check for unusual patterns in CAPTCHA submissions and take action if you notice any abuse or suspicious behavior.
By following these steps and tips, you’ve successfully fortified your Django forms against spam bots and improved the overall security of your website.